Devlog #2 - Main.py issues fix
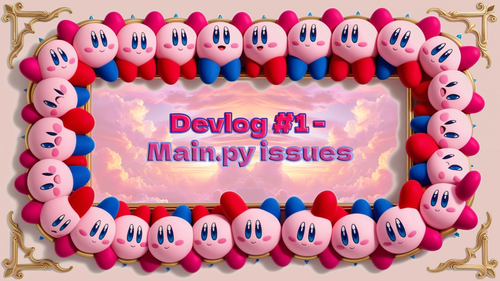
The following is some of the major issues I was able to find thanks to copy and pasting the code from the "Main.py" source file directly into ChatGPT.
The code you provided is well-structured, but there are some potential issues and improvements that could be made:
1. Opening High Score File in Write Mode:
In the __init__
method, you're opening the high score file in write mode ('w'
):
The problem is that opening a file in 'w'
mode will truncate the file (i.e., delete its contents), meaning you’ll always lose the previous high score. You should use 'r'
(read mode) to load the high score, and if the file doesn’t exist, handle it with an exception. For example:
2. Unnecessary Comments on Sprites (Lines 8-9):
You define a sprites
import on line 8, but the actual sprite classes are not shown in the code provided. Ensure that the sprites.py
file exists and contains the necessary sprite classes. Otherwise, this will cause a ModuleNotFoundError
.
3. Sprite Collisions and Health Deduction Logic:
When the player’s health is reduced to 0 or below, you call pg.mixer.music.stop()
and play the death sound, but you don’t properly handle stopping the game loop after the player dies:
This works, but you should ensure that the game doesn't continue processing unnecessary updates after the player dies.
4. Missing Variables and Constants:
PLATFORM_LIST
,SPRITESHEET
,SCREEN_WIDTH
,SCREEN_HEIGHT
,HS_FILE
,TITLE
,FONT_NAME
, and other constants are not defined in the code provided.- The player's health (
PLAYER_HEALTH
),HEALTH_GAIN
,KNOCK_BACK
,SPIKE_DAMAGE
, and other constants related to health and gameplay mechanics are undefined.
Make sure these constants are properly declared in your code before being used. You might want to define them globally at the top of the script, or import them from another module.
5. File Loading and Paths:
Ensure the img
, snd
, and highscore.txt
files exist in the proper directories. If the program runs in a different directory than expected, it may fail to load these files. To ensure cross-platform compatibility, use path.join()
for every file and directory path as you do.
6. Handling Sounds and Music:
In the initialization of sounds, you are directly loading the sounds:
Make sure these files are present in the specified directory, or handle missing sound files with exceptions or fallback.
7. Efficiency in Drawing and Updating:
- In the
update
method, this part of the code continuously increases the player’s y position when it reaches the top 1/4 of the screen:
- Be careful with this, as constantly adjusting sprite positions could lead to unexpected behavior or floating-point precision errors. Ensure the scroll speed is appropriate for smooth gameplay.
8. Comments for Clarity:
While the code is fairly well-commented, consider adding more detailed comments, especially in complex logic parts like collision detection, scoring, and power-up management, to make the code easier to follow.
9. random.choice
import:
While you imported random
, you didn’t explicitly import choice
. Either import it directly or access it via random.choice()
:
10. Uncommented Code for Spawning Enemies:
In the update
method, you have the following commented-out code:
If enemy spawning logic is required, make sure to uncomment and fix this block.
11. Redundant Jump Handling:
The jump logic is handled both in the events()
and the update()
method. You could optimize how you handle this to avoid redundant checks.
Suggestions:
- Test the Game: Try running the game with all assets (sounds, images) and test for crashes or glitches.
- Add Debugging Logs: If the game crashes or behaves unexpectedly, adding logging might help identify issues faster.
Summary of Fixes:
- Change file mode for high score file to
'r'
. - Ensure all necessary constants and imports are properly defined.
- Improve sprite collision and health deduction handling.
- Test file paths and ensure all resources (images, sounds) are accessible.
- Refactor redundant or commented-out code.
With these fixes, your game should function more smoothly!
Get Kirby Super Jump
Kirby Super Jump
Vertical Endless Platformer Featuring Kirby
Status | Prototype |
Author | John Biswas |
Genre | Platformer, Adventure |
Tags | 2D, Casual, Cute, Pixel Art, Singleplayer, Sprites |
More posts
- Devlog #1 - Goals and Next StepsSep 09, 2024
- Project PostmortemSep 08, 2024
Leave a comment
Log in with itch.io to leave a comment.